Space Adventure is a simple text adventure in Python.
The users find themselves, bewildered, inside a drifting spaceship, awakened by an alarm siren; through multiple choices and based on their skills, they have to find a way to prevent the autodestruction of the structure and find a way out.
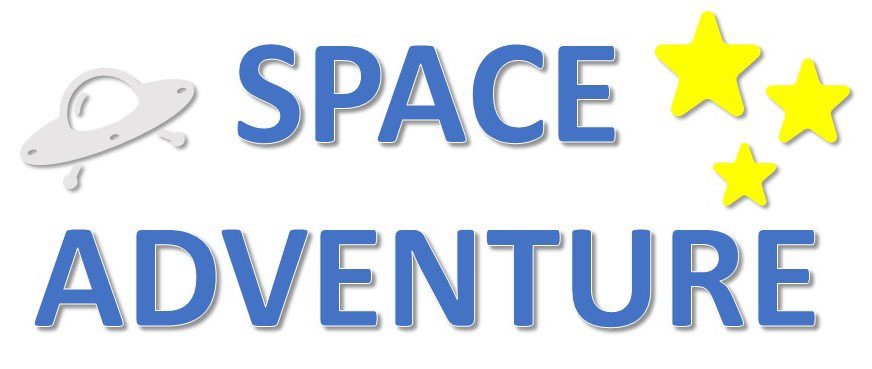
Through the usage of dynamic prompts, functions, and dictionaries, the user will be able to tackle slightly different adventures during every run.
A randomly assigned hero gives the player different stats and tools. Every hero has a different chance to be selected, based on its stats. The statistics of the hero influence the results in battles, the time that every action requires, and in general any roll of the dice.
Every action has a different impact on the countdown, which is calculated at the entrance of every room and may bring to the premature death of the hero.
Equipment and weapons can be found around the spaceships and will be fundamental to find a way out.
To shut down the game, in case the user decides not to try again, I used sys.exit(). This solution is not elegant and badly impacts the perception of the user, but it works at any point the user would like.
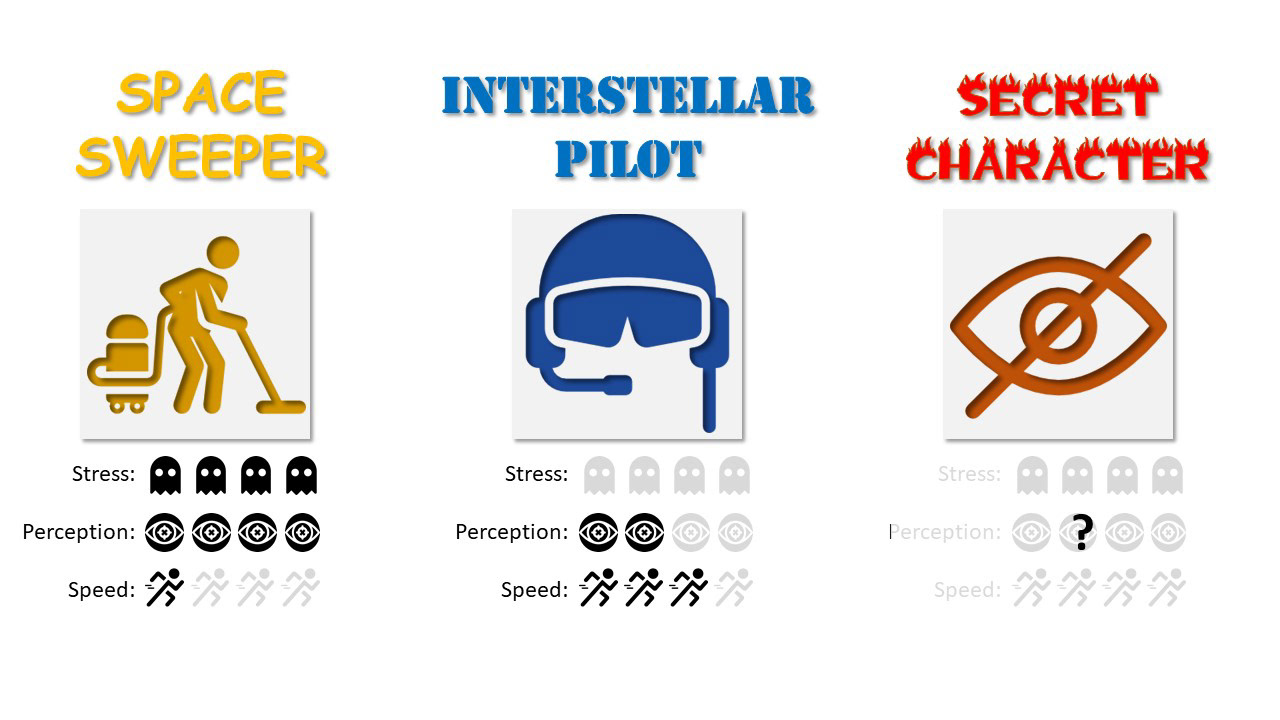
The following code, available also on my Github profile, contains the whole adventure.
#In this first section we import and create all the objects and functions we'll need for the game to work
#Here we input all the packages we need for 1) interrupt the game, 2) choose randomly from a list
import random
import sys
#INTRO------------------------------------------------------------------------
#The introduction to the game
#It contains all the default variable and let the player introduce itself
def intro():
#list of possible characters and their specs
sweeper = {'stress': 4,'perception': 10,'speed': 0.8, 'weapon':"",
'name':'Space Sweeper','health':0}
pilot = {'stress': 0,'perception': 3,'speed': 1.4,'weapon':"wrench",
'name': 'Interstellar Pilot','health':0}
doomguy= {'stress': -10,'perception': 2,'speed': 1,'weapon':"",
'name':'Doomguy','health':0}
#The more redundant the hero the more likely it is to be randomly choosen
hero_list=(sweeper, sweeper, sweeper,sweeper, pilot, pilot, pilot, doomguy)
#set the countdown to 4 minutes
countdown = 60*4
#textual introduction + name question
print("""Your head hurts, a lot.
You open your eyes and look around, but an alarm siren keeps you from putting \
together your thoughts: “where am I?” you think.
....
"I don't even know what the hell is my name"
#list of possible characters and their specs
sweeper = {'stress': 4,'perception': 10,'speed': 0.8, 'weapon':"",
'name':'Space Sweeper','health':0}
pilot = {'stress': 0,'perception': 3,'speed': 1.4,'weapon':"wrench",
'name': 'Interstellar Pilot','health':0}
doomguy= {'stress': -10,'perception': 2,'speed': 1,'weapon':"",
'name':'Doomguy','health':0}
#The more redundant the hero the more likely it is to be randomly choosen
hero_list=(sweeper, sweeper, sweeper,sweeper, pilot, pilot, pilot, doomguy)
#set the countdown to 4 minutes
countdown = 60*4
#textual introduction + name question
print("""Your head hurts, a lot.
You open your eyes and look around, but an alarm siren keeps you from putting \
together your thoughts: “where am I?” you think.
....
"I don't even know what the hell is my name"
""")
print("""
What is your name?""")
name = input(prompt = ">")
print("--------------------------------------------------------------------------")
#we randomly assign the hero and its skills
hero= random.choice(hero_list)
print(f"""
A brief look at yourself confirm your names: your badge states {name}, {hero['name']}.
""")
input("Press any key")
print("""
What is your name?""")
name = input(prompt = ">")
print("--------------------------------------------------------------------------")
#we randomly assign the hero and its skills
hero= random.choice(hero_list)
print(f"""
A brief look at yourself confirm your names: your badge states {name}, {hero['name']}.
""")
input("Press any key")
room_1(countdown,hero)
#"""
#ROOM 1-----------------------------------------------------------------------
#-----------------------------------------------------------------------------
#"""
#ROOM 1-----------------------------------------------------------------------
#-----------------------------------------------------------------------------
def room_1(countdown,hero):
print("""
After a minute or two you are able to focus on the room you are standing \
in: metallic walls, ceiling and floor; it looks like the aseptic interior \
of a spaceship.
A flashing red light attracts your attention: attached to the wall, a digital \
screen is showing a countdown.
“What is going on here?”
print("""
After a minute or two you are able to focus on the room you are standing \
in: metallic walls, ceiling and floor; it looks like the aseptic interior \
of a spaceship.
A flashing red light attracts your attention: attached to the wall, a digital \
screen is showing a countdown.
“What is going on here?”
And, as an answer to your thoughts, a voice, coming from all around you, \
states:""")
#This prints will format the text in a different way
print("\033[40m")
print("\033[1m")
print("\033[97m")
print("""“4 minutes to the auto-destruction. All the crewmembers go to the emergency \
shuttles. There are
…
ZERO
…
available shuttles”.""")
#text format back to normal
print("\033[0m")
print("""
While a pressing sense of urgency grips your stomach, you rapidly look around \
and see a dark hallway in front of you.
What do you do?
""")
#We need a counter to make the action "look around" change its results every time
start_loop=0
#The following function is a finite loop made through a function that recalls itself
look_around_loop(start_loop,countdown,hero)
states:""")
#This prints will format the text in a different way
print("\033[40m")
print("\033[1m")
print("\033[97m")
print("""“4 minutes to the auto-destruction. All the crewmembers go to the emergency \
shuttles. There are
…
ZERO
…
available shuttles”.""")
#text format back to normal
print("\033[0m")
print("""
While a pressing sense of urgency grips your stomach, you rapidly look around \
and see a dark hallway in front of you.
What do you do?
""")
#We need a counter to make the action "look around" change its results every time
start_loop=0
#The following function is a finite loop made through a function that recalls itself
look_around_loop(start_loop,countdown,hero)
#"""
#ROOM 1 LOOK AROUND-----------------------------------------------------------
#-----------------------------------------------------------------------------
#Will follow 2 functions
#A loop that changes (with a limit) the possibilities to choose from whenever you decide to look around
#The more you look around the more you see around you!
#Every "look around" will add a +1 to the loop
def look_around_loop(loop,countdown,hero):
#A series of wrong input results
wrong_prompt=("Your thoughts are not that clean, you are wasting your time",
"What are you blathering? there is no time to waste",
"While you are wasting your time, some really bad things may be happening around",
"Come on! take a proper decision, you don't have much time",
"Time to take a proper decision, come on")
if loop == 0:
print("""
1) Look around a little more
2) Run as fast as you can through the hallway
3) Walk into the hallway
""")
choice = input(prompt=">")
print("--------------------------------------------------------------------------")
#Here and everywhere, all the inputs are compared to a list of possible input from the user, not only the number
#all the letters will be in lower case
if choice.lower() in ("2","run", "hallway",
"fast","run as fast as you can",
"run as fast as you can through the hallway"):
hall_run(countdown,hero)
elif choice.lower() in ("3", "walk","walk into the hallway"):
hall_walk(countdown,hero)
elif choice.lower() in ("1", "look","look around","look around a little more"):
#Every action will result in a reduction of the countdown, divided by the hero speed
countdown = countdown - 15/hero['speed']
loop = loop + 1
look_around_loop(loop,countdown,hero)
else:
#Typos and exceptions won't increase the counter
countdown = countdown - 5/hero['speed']
print(random.choice(wrong_prompt)) #take a random phrase for the wrong list
look_around_loop(loop,countdown,hero)
if loop == 1:
print("""
You look around a little bit more and you notice, on your left, a big handle \
on what seems to be an exit door to the open space.
What do you do?""")
print("""
1) Look around a little more
2) Run as fast as you can through the hallway
3) Walk into the hallway
4) Try to open the door
""")
choice = input(prompt=">")
print("--------------------------------------------------------------------------")
if choice.lower() in ("2","run", "hallway", "fast",
"run as fast as you can",
"run as fast as you can through the hallway"):
hall_run(countdown,hero)
elif choice.lower() in ("3", "walk","walk into the hallway"):
hall_walk(countdown,hero)
elif choice.lower() in ("4", "try","door","try to open the door",
"open the door"):
open_door(countdown,hero)
elif choice.lower() in ("1", "look","look around",
"look around a little more"):
countdown = countdown - 10/hero['speed']
loop = loop + 1
look_around_loop(loop,countdown,hero)
else:
countdown = countdown - 5/hero['speed']
print(random.choice(wrong_prompt)) #take a random phrase for the wrong list
look_around_loop(loop,countdown,hero)
else:
#It's a roll of a dice that will decide if the user will see the hatch
#The possibilities are increased by the perception of the hero
rand_look = random.random()*100 + 5*hero['perception']
if rand_look > 50:
final_look_around(countdown,hero)
else:
print("""
You do not notice anything else particular in the room, even though it’s \
difficult to clearly see around you with that flashing red light that blinds \
you intermittently.
What do you do?""")
print("""
1) Look around a little more
2) Run as fast as you can through the hallway
3) Walk into the hallway
4) Try to open the door
""")
choice = input(prompt=">")
print("--------------------------------------------------------------------------")
if choice.lower() in ("2","run", "hallway", "fast",
"run as fast as you can",
"run as fast as you can through the hallway"):
hall_run(countdown,hero)
elif choice.lower() in ("3", "walk","walk into the hallway"):
hall_walk(countdown,hero)
elif choice.lower() in ("4", "try","door","try to open the door",
"open the door"):
open_door(countdown,hero)
elif choice.lower() in ("1", "look","look around",
"look around a little more"):
countdown = countdown - 20/hero['speed']
look_around_loop(countdown,hero)
else:
countdown = countdown - 5/hero['speed']
print(random.choice(wrong_prompt)) #take a random phrase for the wrong list
look_around_loop(countdown,hero)
#A final "look around" function when nothing can be discovered anymore
def final_look_around(countdown,hero):
print("""
Squinting and focusing on the walls, you notice a small hatch on your right. \
It’s small but you probably would fit in there if you’ll be able to open the \
grill, even though you have no reason to enter.
don’t you?
What do you do?""")
print("""
1) Look around a little more
2) Run as fast as you can through the hallway
3) Walk into the hallway
4) Try to open the exit door
5) Try to enter the hatch
""")
choice = input(prompt=">")
print("--------------------------------------------------------------------------")
if choice.lower() in ("2","run", "hallway", "fast","run as fast as you can",
"run as fast as you can through the hallway"):
hall_run(countdown,hero)
elif choice.lower() in ("3", "walk","walk into the hallway"):
hall_walk(countdown,hero)
elif choice.lower() in ("4","door","try to open the door", "open the door"):
open_door(countdown,hero)
elif choice.lower() in ("1", "look","look around","look around a little more"):
countdown = countdown - 30/hero['speed']
print("""while you continue looking around, an overwhelming sense of \
panic tighten your stomach.
You start running""")
hall_run(countdown,hero)
elif choice.lower() in ("5", "try to enter","enter","hatch","try to enter the hatch"):
hatch(countdown,hero)
else:
print(random.choice(wrong_prompt)) #take a random phrase for the wrong list and reduce the loop
countdown = countdown - 20/hero['speed']
print("""
While you continue looking around, an overwhelming sense of panic tighten \
your stomach.
You start running""")
hall_run(countdown,hero)
on what seems to be an exit door to the open space.
What do you do?""")
print("""
1) Look around a little more
2) Run as fast as you can through the hallway
3) Walk into the hallway
4) Try to open the door
""")
choice = input(prompt=">")
print("--------------------------------------------------------------------------")
if choice.lower() in ("2","run", "hallway", "fast",
"run as fast as you can",
"run as fast as you can through the hallway"):
hall_run(countdown,hero)
elif choice.lower() in ("3", "walk","walk into the hallway"):
hall_walk(countdown,hero)
elif choice.lower() in ("4", "try","door","try to open the door",
"open the door"):
open_door(countdown,hero)
elif choice.lower() in ("1", "look","look around",
"look around a little more"):
countdown = countdown - 10/hero['speed']
loop = loop + 1
look_around_loop(loop,countdown,hero)
else:
countdown = countdown - 5/hero['speed']
print(random.choice(wrong_prompt)) #take a random phrase for the wrong list
look_around_loop(loop,countdown,hero)
else:
#It's a roll of a dice that will decide if the user will see the hatch
#The possibilities are increased by the perception of the hero
rand_look = random.random()*100 + 5*hero['perception']
if rand_look > 50:
final_look_around(countdown,hero)
else:
print("""
You do not notice anything else particular in the room, even though it’s \
difficult to clearly see around you with that flashing red light that blinds \
you intermittently.
What do you do?""")
print("""
1) Look around a little more
2) Run as fast as you can through the hallway
3) Walk into the hallway
4) Try to open the door
""")
choice = input(prompt=">")
print("--------------------------------------------------------------------------")
if choice.lower() in ("2","run", "hallway", "fast",
"run as fast as you can",
"run as fast as you can through the hallway"):
hall_run(countdown,hero)
elif choice.lower() in ("3", "walk","walk into the hallway"):
hall_walk(countdown,hero)
elif choice.lower() in ("4", "try","door","try to open the door",
"open the door"):
open_door(countdown,hero)
elif choice.lower() in ("1", "look","look around",
"look around a little more"):
countdown = countdown - 20/hero['speed']
look_around_loop(countdown,hero)
else:
countdown = countdown - 5/hero['speed']
print(random.choice(wrong_prompt)) #take a random phrase for the wrong list
look_around_loop(countdown,hero)
#A final "look around" function when nothing can be discovered anymore
def final_look_around(countdown,hero):
print("""
Squinting and focusing on the walls, you notice a small hatch on your right. \
It’s small but you probably would fit in there if you’ll be able to open the \
grill, even though you have no reason to enter.
don’t you?
What do you do?""")
print("""
1) Look around a little more
2) Run as fast as you can through the hallway
3) Walk into the hallway
4) Try to open the exit door
5) Try to enter the hatch
""")
choice = input(prompt=">")
print("--------------------------------------------------------------------------")
if choice.lower() in ("2","run", "hallway", "fast","run as fast as you can",
"run as fast as you can through the hallway"):
hall_run(countdown,hero)
elif choice.lower() in ("3", "walk","walk into the hallway"):
hall_walk(countdown,hero)
elif choice.lower() in ("4","door","try to open the door", "open the door"):
open_door(countdown,hero)
elif choice.lower() in ("1", "look","look around","look around a little more"):
countdown = countdown - 30/hero['speed']
print("""while you continue looking around, an overwhelming sense of \
panic tighten your stomach.
You start running""")
hall_run(countdown,hero)
elif choice.lower() in ("5", "try to enter","enter","hatch","try to enter the hatch"):
hatch(countdown,hero)
else:
print(random.choice(wrong_prompt)) #take a random phrase for the wrong list and reduce the loop
countdown = countdown - 20/hero['speed']
print("""
While you continue looking around, an overwhelming sense of panic tighten \
your stomach.
You start running""")
hall_run(countdown,hero)
#DOOR HANDLE------------------------------------------------------------------
#-----------------------------------------------------------------------------
#If the user opens the door will die
#functions for the door on the left
def open_door(countdown,hero):
print("""
As you rotate the handle, the pressure differential tears away the door and \
its handle from you.
You get sucked out from the spaceships and die in the deep space.
The last thing you see is the planet Earth from afar.
It seems so little and vulnerable from here.
""")
die()
#DOOR HANDLE------------------------------------------------------------------
#-----------------------------------------------------------------------------
#the hatch is a secret passage that will let the user win without efforts
def hatch(countdown,hero):
print("""
You try take off the screws by hand, but full of frustration you start \
kicking until it brakes open.
You enter the small passage asking yourself why, if ever, you should get into \
a ventilation pipe.
""")
print("""
The ventilation pipes bring you to the ceiling of a room with little lights \
all over the walls.
You start kicking the grid in front of you to get into the room.
With some efforts the grid falls out and you jump down.
def open_door(countdown,hero):
print("""
As you rotate the handle, the pressure differential tears away the door and \
its handle from you.
You get sucked out from the spaceships and die in the deep space.
The last thing you see is the planet Earth from afar.
It seems so little and vulnerable from here.
""")
die()
#DOOR HANDLE------------------------------------------------------------------
#-----------------------------------------------------------------------------
#the hatch is a secret passage that will let the user win without efforts
def hatch(countdown,hero):
print("""
You try take off the screws by hand, but full of frustration you start \
kicking until it brakes open.
You enter the small passage asking yourself why, if ever, you should get into \
a ventilation pipe.
""")
print("""
The ventilation pipes bring you to the ceiling of a room with little lights \
all over the walls.
You start kicking the grid in front of you to get into the room.
With some efforts the grid falls out and you jump down.
You notice 3 things:
1) The place is actually full of buttons, sreens and lights: it must be some \
kind of control room
2) The grid you just broke felt on some kind of alien creature that was \
roaming around.... and killed it
3) There is a button with written on its side:"Press the red button to avoid \
the destruction of the ship and avoid the fulfillment of the evil plan of a \
previously unknown alien species"
1) The place is actually full of buttons, sreens and lights: it must be some \
kind of control room
2) The grid you just broke felt on some kind of alien creature that was \
roaming around.... and killed it
3) There is a button with written on its side:"Press the red button to avoid \
the destruction of the ship and avoid the fulfillment of the evil plan of a \
previously unknown alien species"
and while you approach the button and save the spaceship from the \
autodestruction you notice a fourth thing:
4) The adventure has been way smoother than expected
""")
input("Press any key")
print("""
YOU SURVIVED AND SAVED THE SHIP!
(it was honestly too easy: you found the secret passage!)
autodestruction you notice a fourth thing:
4) The adventure has been way smoother than expected
""")
input("Press any key")
print("""
YOU SURVIVED AND SAVED THE SHIP!
(it was honestly too easy: you found the secret passage!)
Do you want to try again? [Y/N]""")
#I experimented different kind of loops for unexpected inputs
#Here we have an infinite while loop
while True:
try_again = input(prompt=" >")
if try_again.lower() in ("y","yes","sure"):
print("--------------------------------------------------------------------------")
intro()
break
elif try_again.lower() in ("n","no","never"):
sys.exit("Goodbye")
break
else:
print("Please repeat with yes or no")
#HALLWAY------------------------------------------------------------------
#-----------------------------------------------------------------------------
#the hallway where we may find a wrench, useful during the combat
#running or walking will result in different impacts on the stats of the hero and on the countdown
def hall_run(countdown,hero):
countdown = countdown -15/hero['speed']
hero['stress'] = hero['stress'] + 1
hero['health'] = hero['health'] - 1.5
print("""
You don’t see much, but the countdown wasn’t comforting. You need to get out \
of there, quickly!
As you ran into the dark hallway, you bump into something, and fell ruinously \
on the ground.
#Here we have an infinite while loop
while True:
try_again = input(prompt=" >")
if try_again.lower() in ("y","yes","sure"):
print("--------------------------------------------------------------------------")
intro()
break
elif try_again.lower() in ("n","no","never"):
sys.exit("Goodbye")
break
else:
print("Please repeat with yes or no")
#HALLWAY------------------------------------------------------------------
#-----------------------------------------------------------------------------
#the hallway where we may find a wrench, useful during the combat
#running or walking will result in different impacts on the stats of the hero and on the countdown
def hall_run(countdown,hero):
countdown = countdown -15/hero['speed']
hero['stress'] = hero['stress'] + 1
hero['health'] = hero['health'] - 1.5
print("""
You don’t see much, but the countdown wasn’t comforting. You need to get out \
of there, quickly!
As you ran into the dark hallway, you bump into something, and fell ruinously \
on the ground.
On your knees, you try to find out what made you fall and, groping around, \
you find a crate full of wrenches.
Who abandoned it there must have left in a hurry.
You don’t know what can be waiting for you in the next room.
You collect one of the biggest wrenches.
you find a crate full of wrenches.
Who abandoned it there must have left in a hurry.
You don’t know what can be waiting for you in the next room.
You collect one of the biggest wrenches.
Just in case.
""")
hero['weapon'] = "wrench"
room_2(countdown,hero)
""")
hero['weapon'] = "wrench"
room_2(countdown,hero)
def hall_walk(countdown,hero):
countdown = countdown -30/hero['speed']
print("""
Even though the countdown was not comforting, it does not make sense to take\
the risk of running into the dark and probably getting hurt.
While you walk, you realize a crate full of wrenches has been left there and \
you surely would have hit it running.
What do you do?""")
print("""
1) Take a wrench
2) Continue walking""")
choice = input(prompt=">")
print("--------------------------------------------------------------------------")
if choice.lower() in ("1","take","wrench","take a wrench","take the wrench"):
countdown = countdown -5/hero['speed']
hero['weapon'] = "wrench"
print("""
You don’t know what can be waiting for you in the next room.
You collect one of the biggest wrenches.
countdown = countdown -30/hero['speed']
print("""
Even though the countdown was not comforting, it does not make sense to take\
the risk of running into the dark and probably getting hurt.
While you walk, you realize a crate full of wrenches has been left there and \
you surely would have hit it running.
What do you do?""")
print("""
1) Take a wrench
2) Continue walking""")
choice = input(prompt=">")
print("--------------------------------------------------------------------------")
if choice.lower() in ("1","take","wrench","take a wrench","take the wrench"):
countdown = countdown -5/hero['speed']
hero['weapon'] = "wrench"
print("""
You don’t know what can be waiting for you in the next room.
You collect one of the biggest wrenches.
Just in case.""")
else:
print("You decide to ignore the wrench and continue walking to the \
end of the hallway")
room_2(countdown,hero)
#ROOM 2------------------------------------------------------------------
#-----------------------------------------------------------------------------
#in the room 2 the user will find a robot
#Talking with the robot will result in the access to a bonus room
else:
print("You decide to ignore the wrench and continue walking to the \
end of the hallway")
room_2(countdown,hero)
#ROOM 2------------------------------------------------------------------
#-----------------------------------------------------------------------------
#in the room 2 the user will find a robot
#Talking with the robot will result in the access to a bonus room
def room_2(countdown,hero):
#check if the countdown has reached zero
may_be_late(countdown)
print("""
You are not yet gotten out from the dark of the hallway that a voice state:""")
#shows the coutdown to the user
announcer(countdown)
print("""
As you enter the room, the lights turn on.
A massive robot on crawlers raise its head and its arms as a marionette and \
start approaching you.
A white light comes out of its eyes.
What do you do?""")
print("""
1) Fight the robot
2) Present yourself to the robot
""")
choice = input(prompt=">")
print("--------------------------------------------------------------------------")
if choice.lower() in ("1","fight","fight the robot","i hate the damned robot"):
print("""You attack first.
If possible, the robot seems surprised by your attack and this gives \
you advantage.
""")
#the fight has various results based on random values and hero stats
#The possible outputs are: victory, victory with stats decrease, death
fight_result = random.random()*100 + hero['health']*10
if hero['weapon'] == "wrench":
fight_result = fight_result +20
#Doomguy won't lose any battle
if hero['name'] == "Doomguy":
fight_result = 100
if fight_result < 30:
print("""
You hit the robot first, but you barely scratch its coating.
The robot’s eyes turn red and he starts fighting back and with its metallic \
arms takes you down.
With a feral rage, heavy as a tank, it drives on you with its crawlers.
You die.
""")
die()
elif fight_result < 90:
countdown = countdown -100/hero['speed']
hero['healt'] = hero['health'] -4
hero['stress'] = hero['stress'] +3
print("""
After your first successful strike on its head, the robot starts reacting: \
its eyes turn red and it starts rotating its harms rapidly in your direction.
It hits your chest and an explosion of pain invade your body.
You fall on the ground, but the robot continues to blindly hit the air in \
front of it.
You must have damaged its sensors.
You creep out of the room through another corridor: it’s too dangerous to get \
close again to that metallic monster.""")
room_3(countdown,hero)
else:
countdown=countdown-30/hero['speed']
hero['stress'] = hero['stress'] -2
print("""
Your first hit on the head of the robot is incredibly successful: the lights \
in the eyes of the robot turns off and it collapses helpless.
Proud of yourself and your strength, you walk out of the room through another \
corridor""")
room_3(countdown,hero)
elif choice.lower in ("2","present yourself to the robot","present",
"present yourself"):
countdown = countdown -40/hero['speed']
print("""
As the robot get closer, its eyes turn from white to blue and it starts talking:
“Hello there, space traveler!
I am KRN4449RT-bis and I am here to make your spaceflight the most pleasing \
as possible!
I am sorry to inform you that while you were crio-sleeping, Bob, a member of \
the crew, driven crazy by an unknown alien species, has killed every other \
human being here and has planned the destruction of the ship. Unfortunately, \
nobody knows the real reasons behind his actions but, in order to make your \
spaceflight the most pleasing as possible, I have to make sure that this \
spaceship won’t explode in the next few minutes. you need to stop Bob and \
his evil plan. Just push the big red button in the control room to stop the \
countdown!”
“In order to make your adventure more pleasing, I have to advise you to get \
some military equipment in the armory.
The password to enter is “Password” “
You thank the robot and, confused about the situation you found yourself, you \
walk through the next hallway
""")
input("Press any key")
else:
countdown = countdown -50/hero['speed']
print("""
As the robot get closer, and you still haven't made your mind, its eyes turn \
from white to blue and it starts talking:
“Hello there, space traveler!
I am KRN4449RT-bis and I am here to make your spaceflight the most pleasing \
as possible!
I am sorry to inform you that while you were crio-sleeping, Bob, a member of \
the crew, driven crazy by an unknown alien species, has killed every other \
human being here and has planned the destruction of the ship. Unfortunately, \
nobody knows the real reasons behind his actions but, in order to make your \
spaceflight the most pleasing as possible, I have to make sure that this \
spaceship won’t explode in the next few minutes. you need to stop Bob and \
his evil plan. Just push the big red button in the control room to stop the \
countdown!”
“In order to make your adventure more pleasing, I have to advise you to get \
some military equipment in the armory.
The password to enter is “Password” “
You thank the robot and, confused about the situation you found yourself, \
you walk through the next hallway
""")
room_3(countdown,hero)
#ROOM 3------------------------------------------------------------------
#-----------------------------------------------------------------------------
#The room 3 is a central node for many outcomes that will lead to death, a bonus room or the final battle
def room_3(countdown,hero):
#A series of wrong input results
wrong_prompt_2=("Stars are wonderful, but please, there is no time to waste!!",
"What's wrong with you?", "Your thoughts are still not in order",
"You seem a little bit confused, don't you?")
may_be_late(countdown)
print("Again, the spaceship AI shouts on the speakers:")
announcer(countdown)
countdown = countdown - 30/hero['speed']
print("""
Entering the room, you find yourself in front of a huge glass window on the \
deep space.
The panorama is mesmerizing.
This room must be a central node of the spaceship: many corridors crowd the \
walls around the window.
Each corridor is labeled.
Where do you want to go?
""")
print("""“Armory”, “Shuttles”, “Wing 1”, “Wing 2”, “Dorms”, “Engines”,
“Control room”""")
choice = input(prompt=">")
print("--------------------------------------------------------------------------")
if choice.lower() in ("armory","arm"):
armory(countdown,hero)
elif choice.lower() in ("wing","wing 1", "wing 2","wing1","wing2",
"wings", "dorm","dorms",
"engine","engines"):
corridors(countdown,hero,choice)
elif choice.lower() in ("shuttle","shuttles"):
print("""
As you walk through the corridors you remember the alarm voice telling you \
that there were zero shuttles available.
Your fears materialize when you get to the dock and don’t find any available \
shuttle.
You start crying, in despair.
""")
too_late()
elif choice.lower() in ("control","controlroom","control room","room"):
control_room(countdown,hero)
else:
print(random.choice(wrong_prompt_2)) #take a random phrase from the wrong list
room_3(countdown,hero)
wrong_prompt_2=("Stars are wonderful, but please, there is no time to waste!!",
"What's wrong with you?", "Your thoughts are still not in order",
"You seem a little bit confused, don't you?")
may_be_late(countdown)
print("Again, the spaceship AI shouts on the speakers:")
announcer(countdown)
countdown = countdown - 30/hero['speed']
print("""
Entering the room, you find yourself in front of a huge glass window on the \
deep space.
The panorama is mesmerizing.
This room must be a central node of the spaceship: many corridors crowd the \
walls around the window.
Each corridor is labeled.
Where do you want to go?
""")
print("""“Armory”, “Shuttles”, “Wing 1”, “Wing 2”, “Dorms”, “Engines”,
“Control room”""")
choice = input(prompt=">")
print("--------------------------------------------------------------------------")
if choice.lower() in ("armory","arm"):
armory(countdown,hero)
elif choice.lower() in ("wing","wing 1", "wing 2","wing1","wing2",
"wings", "dorm","dorms",
"engine","engines"):
corridors(countdown,hero,choice)
elif choice.lower() in ("shuttle","shuttles"):
print("""
As you walk through the corridors you remember the alarm voice telling you \
that there were zero shuttles available.
Your fears materialize when you get to the dock and don’t find any available \
shuttle.
You start crying, in despair.
""")
too_late()
elif choice.lower() in ("control","controlroom","control room","room"):
control_room(countdown,hero)
else:
print(random.choice(wrong_prompt_2)) #take a random phrase from the wrong list
room_3(countdown,hero)
#The user may come back in room 3, we don't want all the description again
def room_3_again(countdown,hero):
may_be_late(countdown)
print("""
Again, the spaceship AI shouts on the speakers:""")
announcer(countdown)
print("""
You are again in the window room.
What now?
“Armory”, “Shuttles”, “Wing 1”, “Wing 2”, “Dorms”, “Engines”, “Control room”
""")
choice = input(prompt=">")
print("--------------------------------------------------------------------------")
if choice.lower() in ("armory","arm"):
armory(countdown,hero)
elif choice.lower() in ("wing","wing 1", "wing 2","wing1","wing2",
"wings", "dorm","dorms",
"engine","engines"):
corridors(countdown,hero,choice)
elif choice.lower() in ("shuttle","shuttles"):
print("""
As you walk through the corridors you remind the alarm voice telling you that \
there were zero shuttles available.
Your fears materialize when you get to the dock and don’t find any available \
shuttle.
You start crying, in despair.
""")
too_late()
elif choice.lower() in ("control","controlroom","control room","room"):
control_room(countdown,hero)
else:
print("You probably lost enough time, you enter the control room") #take a random phrase for the wrong list
control_room(countdown,hero)
#CORRIDORS--------------------------------------------------------------------
#-----------------------------------------------------------------------------
#In the corridors the user can get lost and die or may find his/her way back
def corridors(countdown,hero,place):
#Doomguy cannot get lost
if hero['name']=="Doomguy":
print(f"""
After wasting your time walking in corridoors full of dead bodies, \
looking for {place.lower()} and blood, you come back to the main room, ready to enter the control room""")
countdown=countdown - 100/hero['speed']
room_3_again(countdown,hero)
else:
print(f"""
For no good reason you decide to explore the spaceship and visit the {place.lower()}.
After the first turn of the corridor you see a dead body on the ground, blood and flesh all over the walls.
Terrified, you start running until you get lost in an infernal maze of hallways and doors.
Dead bodies everywhere, and horrific sounds from the dark.
""")
#getting lost or coming back is a randomly choosen
corridor_result = random.random()*100
if corridor_result < 50:
print("""You try to get back from where you came but you continue \
finding yourself surrounded by horrifying sceneries, until the countdown reaches the zero.""")
too_late()
else:
countdown= countdown -100/hero['speed']
hero['stress']=hero['stress'] + 3
print("""
Out of breath, but full of luck and fear, you succeed in gettin back to the main room.
There is no more time to waste, you should go to the control room.
""")
room_3_again(countdown,hero)
#ARMORY----------------------------------------------------------------------
#-----------------------------------------------------------------------------
#We can access the armory only after a talk with the robot
#Every weapon will lead to different output in the final battle
#The password input loop is done through a function that calls itself
def armory(countdown,hero):
countdown = countdown - 10/hero['speed']
print("""The door can be opened only through a code:""")
print("""
Insert the code or (2) go back
""")
choice = input(prompt=">")
print("--------------------------------------------------------------------------")
if choice.lower() == "password":
print("""
The door opens.
Shelfs full of weapons and cartridges in front of your eyes, all this \
firepower encourage you: nothing will be able to stop you now.""")
hero['stress'] = hero['stress']-3
countdown=countdown - 25/hero['speed']
in_armory(countdown,hero)
elif choice.lower() in ("2","(2)","back","go","go back"):
room_3_again(countdown,hero)
else:
print("Wrong password")
armory(countdown,hero)
firepower encourage you: nothing will be able to stop you now.""")
hero['stress'] = hero['stress']-3
countdown=countdown - 25/hero['speed']
in_armory(countdown,hero)
elif choice.lower() in ("2","(2)","back","go","go back"):
room_3_again(countdown,hero)
else:
print("Wrong password")
armory(countdown,hero)
#here we get into the armory
def in_armory(countdown,hero):
countdown=countdown - 5/hero['speed']
print("""
Choose a weapon:
""")
print("""
1) Plasma machine-gun
2) Lightsaber
3) Nuclear bazooka
""")
weapon_chosen= input(prompt=">")
print("--------------------------------------------------------------------------")
if weapon_chosen.lower() in ("1","plasma","machine-gun","gun",
"plasma machine-gun", "machine gun",
"plasma machine gun", "machinegun",
"plasma machinegun"):
hero['weapon'] = "plasma machine-gun"
elif weapon_chosen.lower() in ("2","light","saber","lightsaber",
"i wanna be a jedi!"):
hero['weapon'] = "lightsaber"
elif weapon_chosen.lower() in ("3","nuclear","bazoka","bazooka",
"bazoooka","nuclear bazooka",
"nuclear bazoka"):
hero['weapon'] = "nuclear bazooka"
else:
print("You probably haven't understood what the armory can offer you")
in_armory(countdown,hero)
room_3_again(countdown,hero)
#CONTROL ROOM-----------------------------------------------------------------
#-----------------------------------------------------------------------------
#The control room in which there will be the final battle
def in_armory(countdown,hero):
countdown=countdown - 5/hero['speed']
print("""
Choose a weapon:
""")
print("""
1) Plasma machine-gun
2) Lightsaber
3) Nuclear bazooka
""")
weapon_chosen= input(prompt=">")
print("--------------------------------------------------------------------------")
if weapon_chosen.lower() in ("1","plasma","machine-gun","gun",
"plasma machine-gun", "machine gun",
"plasma machine gun", "machinegun",
"plasma machinegun"):
hero['weapon'] = "plasma machine-gun"
elif weapon_chosen.lower() in ("2","light","saber","lightsaber",
"i wanna be a jedi!"):
hero['weapon'] = "lightsaber"
elif weapon_chosen.lower() in ("3","nuclear","bazoka","bazooka",
"bazoooka","nuclear bazooka",
"nuclear bazoka"):
hero['weapon'] = "nuclear bazooka"
else:
print("You probably haven't understood what the armory can offer you")
in_armory(countdown,hero)
room_3_again(countdown,hero)
#CONTROL ROOM-----------------------------------------------------------------
#-----------------------------------------------------------------------------
#The control room in which there will be the final battle
def control_room(countdown,hero):
countdown=countdown -20/hero['speed']
may_be_late(countdown)
print("Again, the spaceship AI shouts on the speakers:")
announcer(countdown)
print("""
You enter the control room. The sound of the alarm is louder than ever.
There is no more time.
The wall on the opposite side of the room is crowded by lights, screens and \
button, but you are still able to recognize THE Button: a red button \
flashing in the center of the room. A big signal on its side states: \
“Press the red button to avoid the destruction of the ship and avoid the \
fulfillment of the evil plan of a previously unknown alien species”.
As you approach the red button, a disgraceful, disgusting, wrong creature \
blocks your way. It’s Bob.
What do you do?
""")
print("""
1) Attack
2) Say Hi
""")
choice = input(prompt=">")
print("--------------------------------------------------------------------------")
if choice.lower() in ("say","hi","say hi", "2"):
print("""
You wave your hand at Bob.
button, but you are still able to recognize THE Button: a red button \
flashing in the center of the room. A big signal on its side states: \
“Press the red button to avoid the destruction of the ship and avoid the \
fulfillment of the evil plan of a previously unknown alien species”.
As you approach the red button, a disgraceful, disgusting, wrong creature \
blocks your way. It’s Bob.
What do you do?
""")
print("""
1) Attack
2) Say Hi
""")
choice = input(prompt=">")
print("--------------------------------------------------------------------------")
if choice.lower() in ("say","hi","say hi", "2"):
print("""
You wave your hand at Bob.
Bob seems surprised. He/It retracts its claws and tooth.
“Hey there! I guess you want to stop my evil plan! Why don’t I walk you \
through it?
First of all, I’m not Bob, not anymore, but I don’t think you can even \
pronounce my proper alien name. Just call me Bob, nice to meet you.
I fulfilled the engine room with biogenic alien tanks: when this spaceship \
will explode, a rain of deadly foam will get into the Earth orbit and will \
then transform the whole human race in monsters like me.
“Hey there! I guess you want to stop my evil plan! Why don’t I walk you \
through it?
First of all, I’m not Bob, not anymore, but I don’t think you can even \
pronounce my proper alien name. Just call me Bob, nice to meet you.
I fulfilled the engine room with biogenic alien tanks: when this spaceship \
will explode, a rain of deadly foam will get into the Earth orbit and will \
then transform the whole human race in monsters like me.
I may get in much more details, just to distract you from trying to stop me, \
but I have no need to: the time ended.
but I have no need to: the time ended.
Nice try!” """)
too_late()
elif choice.lower() in ("attack","attak","attack!!!!!!!!!!!", "1"):
attack(countdown,hero) #a function for the attack option: it's a very busy part of code
else:
print("""
While you still write typos on this prompt, Bob attacks you.
You die.""")
die()
#the attack is strongly influenced by the hero, its specs,the weapon and the luck
def attack(countdown,hero):
countdown = countdown - 20/hero['speed']
if hero['name']== "Doomguy":
print("""You don't even need a weapon to defeat Bob: you rip off \
its harm and start hitting him with its own fist.
it doesn't take much time before it collapses to the ground, helpless.
""")
button(countdown,hero)
elif hero['weapon']== "":
print("""You attack barehanded a massive monster.
What did you expect?
You die.
""")
elif hero['weapon']== "wrench":
print("""
You know that you won’t be able to take off a similar monster with just a \
wrench: so you throw the wrench in the direction of the red button.""")
wrench_result = random.random()*100
if wrench_result < 50:
print("""
You miss the red button.
Bob eats you while you blame your poor basketball skills.
You die.
""")
die()
else:
print("""
You hit the button and stop the countdown, seconds before the explosion.
Bob attacks you and kills you.
After you die, Bob simply start the auto-destruction process again and now \
with nobody able to stop it.
""")
die()
elif hero['weapon']== "plasma machine-gun":
print("""You raise your plasma machine gun and aim for the head of \
the monster.""")
plasma_result = random.random()*100
if hero['stress'] > 5:
print("""
Your hands are shaking for all the stress accumulated. You are unable to aim \
properly. You miss it.
Bob eats you.
You die.
""")
die()
elif plasma_result < 50:
print("""You miss it.
Bob eats you.
You die.
""")
else:
print("""Your aim is perfect and the projectile blow the head of \
the monsters.
Alien blood all over the place.
Bob dies.
""")
button(countdown,hero)
elif hero['weapon']== "lightsaber":
print("""You unsheathe your lightsaber like a true jedi and face \
the monster""")
if hero['health'] < -2:
print("""Your body still hurts from the previous fight and your \
mind screams for pain while you attack""")
saber_result = random.random()*100 - 5* hero['health']
if saber_result < 40:
print("""
The monster is faster than you and tears up your harm.
You see your hand still holding the light saber falling on the ground.
Bob approach your face with his jaws.
def attack(countdown,hero):
countdown = countdown - 20/hero['speed']
if hero['name']== "Doomguy":
print("""You don't even need a weapon to defeat Bob: you rip off \
its harm and start hitting him with its own fist.
it doesn't take much time before it collapses to the ground, helpless.
""")
button(countdown,hero)
elif hero['weapon']== "":
print("""You attack barehanded a massive monster.
What did you expect?
You die.
""")
elif hero['weapon']== "wrench":
print("""
You know that you won’t be able to take off a similar monster with just a \
wrench: so you throw the wrench in the direction of the red button.""")
wrench_result = random.random()*100
if wrench_result < 50:
print("""
You miss the red button.
Bob eats you while you blame your poor basketball skills.
You die.
""")
die()
else:
print("""
You hit the button and stop the countdown, seconds before the explosion.
Bob attacks you and kills you.
After you die, Bob simply start the auto-destruction process again and now \
with nobody able to stop it.
""")
die()
elif hero['weapon']== "plasma machine-gun":
print("""You raise your plasma machine gun and aim for the head of \
the monster.""")
plasma_result = random.random()*100
if hero['stress'] > 5:
print("""
Your hands are shaking for all the stress accumulated. You are unable to aim \
properly. You miss it.
Bob eats you.
You die.
""")
die()
elif plasma_result < 50:
print("""You miss it.
Bob eats you.
You die.
""")
else:
print("""Your aim is perfect and the projectile blow the head of \
the monsters.
Alien blood all over the place.
Bob dies.
""")
button(countdown,hero)
elif hero['weapon']== "lightsaber":
print("""You unsheathe your lightsaber like a true jedi and face \
the monster""")
if hero['health'] < -2:
print("""Your body still hurts from the previous fight and your \
mind screams for pain while you attack""")
saber_result = random.random()*100 - 5* hero['health']
if saber_result < 40:
print("""
The monster is faster than you and tears up your harm.
You see your hand still holding the light saber falling on the ground.
Bob approach your face with his jaws.
Bob eats you.
You die.
""")
die()
else:
print("""As Bob tries to attack you, you are able to cut him in \
half. Alien blood all over you.
Bob dies.
""")
if hero['stress'] > 3:
print("""Full of alien blood and monstrous innards, you \
can’t stop yourself from throwing up all over the place""")
button(countdown,hero)
elif hero['weapon']=="nuclear bazooka":
print("""
You raise your nuclear bazooka on your shoulder.
Bob expression changes from ferocious to scared…
While you pull the trigger, Bob starts laughing at you.
The missile reaches Bob and blows up him, the control room, you, and the \
whole spaceship.
Everybody dies.
Whatever it was, the evil plan is fulfilled anyhow.
""")
die()
#BUTTON----------------------------------------------------------------------
#-----------------------------------------------------------------------------
#the victory function
""")
die()
#BUTTON----------------------------------------------------------------------
#-----------------------------------------------------------------------------
#the victory function
def button(countdown, hero):
print("""
You get to the button very few seconds before everything explodes.
As you press the button, the normal lights turn on again, and the alarm stops.
You feel relieved: Bob is dead, and you are finally safe
print("""
You get to the button very few seconds before everything explodes.
As you press the button, the normal lights turn on again, and the alarm stops.
You feel relieved: Bob is dead, and you are finally safe
…
If there’s nothing else haunting the spaceship
…
IF
…
""")
input("Press any key")
print("""
YOU SURVIVED AND SAVED THE SHIP!
input("Press any key")
print("""
YOU SURVIVED AND SAVED THE SHIP!
Do you want to try again? [Y/N]""")
while True:
try_again = input(prompt=" >")
if try_again.lower() in ("y","yes","sure"):
print("--------------------------------------------------------------------------")
intro()
break
elif try_again.lower() in ("n","no","never"):
sys.exit("Goodbye")
break
else:
print("Please answer with yes or no")
#DIE FUNCTION-----------------------------------------------------------------
#-----------------------------------------------------------------------------
#a "die" function for when the player dies and decide wheter to restart or not the game
def die():
print("Press any key")
print("""
try_again = input(prompt=" >")
if try_again.lower() in ("y","yes","sure"):
print("--------------------------------------------------------------------------")
intro()
break
elif try_again.lower() in ("n","no","never"):
sys.exit("Goodbye")
break
else:
print("Please answer with yes or no")
#DIE FUNCTION-----------------------------------------------------------------
#-----------------------------------------------------------------------------
#a "die" function for when the player dies and decide wheter to restart or not the game
def die():
print("Press any key")
print("""
Do you want to play again? [Y/N]""")
while True:
try_again = input(prompt=" >")
if try_again.lower() in ("y","yes","sure"):
print("--------------------------------------------------------------------------")
intro()
break
elif try_again.lower() in ("n","no","never"):
sys.exit("Goodbye")
break
else:
print("Please answer with yes or no")
#COUNTDOWN CHECK--------------------------------------------------------------
#-----------------------------------------------------------------------------
#At the beginning of every room , it checks if the countdown has reached the zero
#It eventually recall the too_late function
def may_be_late(countdown):
if countdown < 0:
too_late()
#ANNOUNCER--------------------------------------------------------------------
#-----------------------------------------------------------------------------
#After the countdown is checked, it will be printed for the user
def announcer(countdown):
input("Press any key")
#This prints will format the text in a different way
print("\033[40m")
print("\033[1m")
print("\033[97m")
print(f"""“
{round(countdown)} seconds to the auto-destruction. All the crewmembers go to \
the emergency shuttles. There are
…
ZERO
…
available shuttles”""")
#text format back to normal
print("\033[0m")
#COUNTDOWN CHECK--------------------------------------------------------------
#-----------------------------------------------------------------------------
#function to end the game when counter goes to zero. It recalls the die function
while True:
try_again = input(prompt=" >")
if try_again.lower() in ("y","yes","sure"):
print("--------------------------------------------------------------------------")
intro()
break
elif try_again.lower() in ("n","no","never"):
sys.exit("Goodbye")
break
else:
print("Please answer with yes or no")
#COUNTDOWN CHECK--------------------------------------------------------------
#-----------------------------------------------------------------------------
#At the beginning of every room , it checks if the countdown has reached the zero
#It eventually recall the too_late function
def may_be_late(countdown):
if countdown < 0:
too_late()
#ANNOUNCER--------------------------------------------------------------------
#-----------------------------------------------------------------------------
#After the countdown is checked, it will be printed for the user
def announcer(countdown):
input("Press any key")
#This prints will format the text in a different way
print("\033[40m")
print("\033[1m")
print("\033[97m")
print(f"""“
{round(countdown)} seconds to the auto-destruction. All the crewmembers go to \
the emergency shuttles. There are
…
ZERO
…
available shuttles”""")
#text format back to normal
print("\033[0m")
#COUNTDOWN CHECK--------------------------------------------------------------
#-----------------------------------------------------------------------------
#function to end the game when counter goes to zero. It recalls the die function
def too_late():
input("Press any key")
#This prints will format the text in a different way
print("\033[40m")
print("\033[1m")
print("\033[97m")
print("""
The intermitting alarm stops.
“Countdown ended.
The spaceship will explode in:
3
2
1”""")
#text format back to normal
print("\033[0m")
print("""The ship explodes.
input("Press any key")
#This prints will format the text in a different way
print("\033[40m")
print("\033[1m")
print("\033[97m")
print("""
The intermitting alarm stops.
“Countdown ended.
The spaceship will explode in:
3
2
1”""")
#text format back to normal
print("\033[0m")
print("""The ship explodes.
You die, and your body - or what remains of it - floats into the space with \
the debris of the spaceship.
the debris of the spaceship.
strange foam expands from the relict for kilometers around the explosion site.
From your perspective - if you could only have one - this foam seems to wrap \
the entire planet Earth: the planet is still far, but somehow on its trajectory.
""")
#text format back to normal
print("\033[0m")
die()
the entire planet Earth: the planet is still far, but somehow on its trajectory.
""")
#text format back to normal
print("\033[0m")
die()
#GAME START-------------------------------------------------------------------
#-----------------------------------------------------------------------------
#-----------------------------------------------------------------------------
intro()